Image Binarization#
When binarizing an image, we produce an image that has only two values: True and False. Often, images are also called if they contain the value 0
, e.g. for background, and any other value for foreground.
import numpy as np
from skimage import data
from skimage.io import imread
from skimage.filters import threshold_otsu
import matplotlib.pyplot as plt
We use this example image of nuclei.
image_nuclei = imread('data/mitosis_mod.tif')
plt.imshow(image_nuclei, cmap='gray')
plt.colorbar()
<matplotlib.colorbar.Colorbar at 0x7f168ed67820>
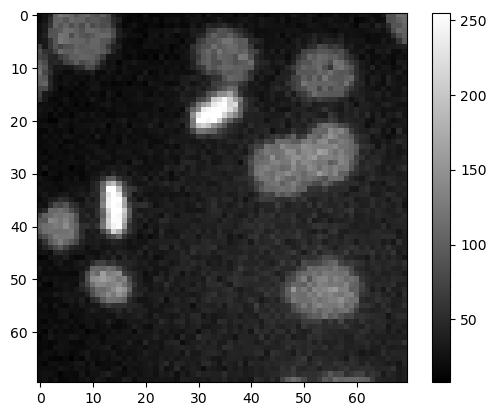
Image Thesholding#
The most common binarization technique is thresholding. We apply a threshold to determine which pixel lie above a certain pixel intensity and which are below.
image_binary = image_nuclei > 125
plt.imshow(image_binary, cmap='gray')
plt.colorbar()
<matplotlib.colorbar.Colorbar at 0x7f168ebac490>
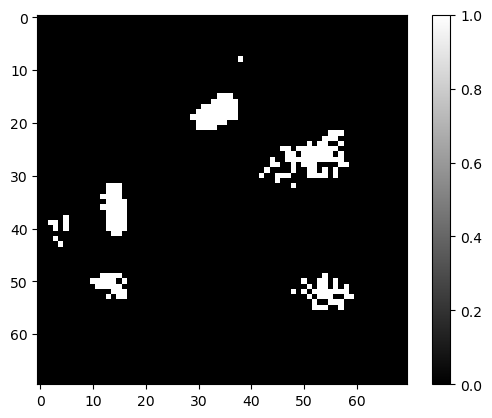
As choosing a manual threshold introduces human bias, it might be better to apply an automated thresholding algorithm such as Otsu’s method.
threshold = threshold_otsu(image_nuclei)
threshold
77
image_otsu_binary = image_nuclei > threshold
plt.imshow(image_otsu_binary, cmap='gray')
plt.colorbar()
<matplotlib.colorbar.Colorbar at 0x7f168ecd78e0>
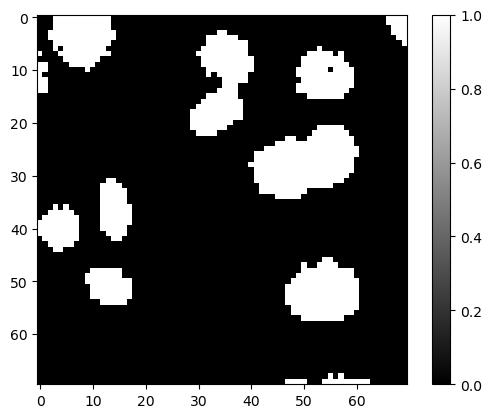